Blog
Modern App Deployment with AWS CodePipeline
Traditional software development typically involves moving code updates manually and infrequently from development to testing and then production. Because of handoffs between several teams along the way, this method is cumbersome, archaic, and slow. As with anything done or handled manually, this technique makes it difficult for developers to consistently release updates and new applications.
The ability to meet the needs of our customers, especially delivering updates and new software, is at the core of every successful business. Companies that can rapidly provide updates and innovate faster can adapt to changing markets quicker, driving better business results.
What’s the trick? Successful companies use automated tools like AWS CodePipeline in their software release process because automation enables testing and releasing code more frequently.
To see how AWS CodePipeline can help us succeed too, let’s look at the following:
- AWS CodePipeline: what it is and what it can do
- The benefits of using AWS CodePipeline
- Continuous integration and continuous delivery (CI/CD) tools and how they ease the transition from monolithic to microservice-based apps
We’ll also go through a hands-on demonstration showing how to create a pipeline that takes a Java microservice (written as an AWS Lambda function) with its code stored in AWS CodeCommit, build it, runs tests on it, and deploy it to AWS Lambda.
Let’s begin!
A Primer for AWS CodePipeline
AWS CodePipeline is a CD service that allows us to automatically deliver, build, test, and deploy our code updates.
Additionally, CodePipeline lets us model, visualize, and automate our software release process, either through a simple graphical user interface or with a command-line interface. It also enables us to model our software release process as a workflow of different stages, or a pipeline that describes how new code changes progress through our release process.
With AWS CodePipeline, we can monitor our code processes in real-time and ensure a consistent release process. We can also speed up delivery while improving quality, and view pipeline history details because monitoring becomes very easy.
AWS CodePipeline, though sometimes overlooked, is every bit as capable as other CI/CD tools that dev and DevOps teams use. More importantly, it offers something they don’t: deep, easy integration with all AWS services where we might want to deploy an application.
What Can We Do with AWS CodePipeline?
We can do the following with AWS CodePipeline:
- We can run a consistent release process. With CodePipeline, we can set predefined rules for code changes. This lets CodePipeline run each stage of our release according to the rules.
- We can hasten delivery. With CodePipeline’s automation model, we can test and release code exponentially as we deem fit and hasten the release of new application features.
- We can view progress on the go. With CodePipeline, we can see in real-time the status of our pipelines on the go, check and view details, retry failed actions, and so on.
- We can view pipeline history details. CodePipeline lets us view the history of a pipeline, such as the start and end times, including run durations.
In the following section, we look at some of the benefits of using AWS CodePipeline.
Benefits of Using AWS CodePipeline
The benefits of using AWS CodePipeline are:
- Decreased development time
- Ease in scaling
- Configurable workflow
AWS CodePipeline reduces development time with its simplified development approach, containing only a few manual steps, and its automated dev and test environments. For example, instead of taking two days to build a new production account, companies can do it in minutes using AWS CloudFormation templates and AWS CodePipeline. This gives us the luxury of quickly launching small-scale projects that cost very little and take very little time to set up. With this structure in place, we can easily experiment and get the best solutions rather than settling for what we have.
Another benefit of using the AWS CodePipeline is that it allows us to quickly scale up or down without acquiring and provisioning our servers months in advance. With AWS, the server-provisioning process takes minutes instead of weeks, which is the case using traditional techniques for scaling. As a result, organizations can also scale faster.
With AWS CodePipeline, we can easily configure the different stages of our software release process using the console interface, AWS CLI, AWS CloudFormation, or the AWS SDKs. We can specify tests to run and customize the steps to deploy our application.
AWS CodePipeline enables us to easily and quickly build application environments and manage our applications using AWS CodeDeploy and CodeCommit. This benefits us by making DevOps and IT teams more efficient.
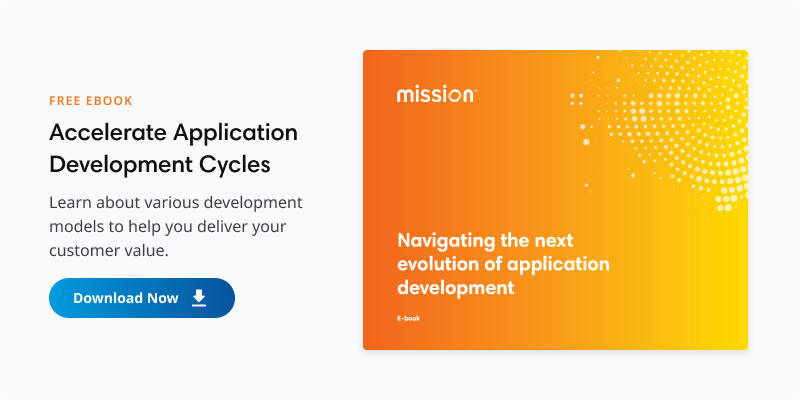
How AWS CodePipeline Eases the Transition from Monolithic to Microservice-based Apps
Let’s discuss how AWS CodePipeline eases the transition from monolithic to microservice-based apps.
A monolithic-based app is a single unit of deployment that handles multiple types of business capability, usually all tightly coupled. In contrast, microservices take each core business capability and deploy it as a separate unit, performing a single function.
AWS CodePipeline helps ease the transition from monolithic to microservice-based apps by breaking down monolithic applications into microservices using decomposition patterns. The decomposition patterns convert them into several microservices.
This approach eases the transition process for the following reasons:
- It provides faster innovation because each microservice can be individually tested and deployed.
- It helps ensure smooth, rapid adjustments to fluctuating business demand without interrupting core activities, such as high scalability, improved resiliency, continuous delivery, and failure isolation.
- It provides an efficient transition of monolithic applications into a microservices architecture.
AWS CodePipeline also helps ease the transition from a monolithic to a microservice app by integrating the newly created microservices into a microservices architecture using AWS serverless services.
Prerequisites
Now that we’ve given an overview of AWS CodePipeline, let’s review a hands-on demonstration of how to create a pipeline that takes a Java microservice (written as an AWS Lambda function) with its code stored in AWS CodeCommit. We’ll see how to build it, run tests on it, and deploy it to AWS Lambda.
For newbies using AWS CodePipeline for the first time, you need to start by completing the following steps:
- Create an AWS account if you don’t have one.
- Follow the instructions to create an IAM user in your AWS account.
- Use an IAM-managed policy to assign CodePipeline permissions to the IAM user.
- Install the AWS CLI.
- Open the console for CodePipeline.
Note: Ensure you save your AWS access key ID and secret access key, as you need them later to sign in to your console.
The screenshot below shows the CodePipeline console that we use to build, run, test, and deploy our Java microservice written as an AWS Lambda function for AWS Lambda.
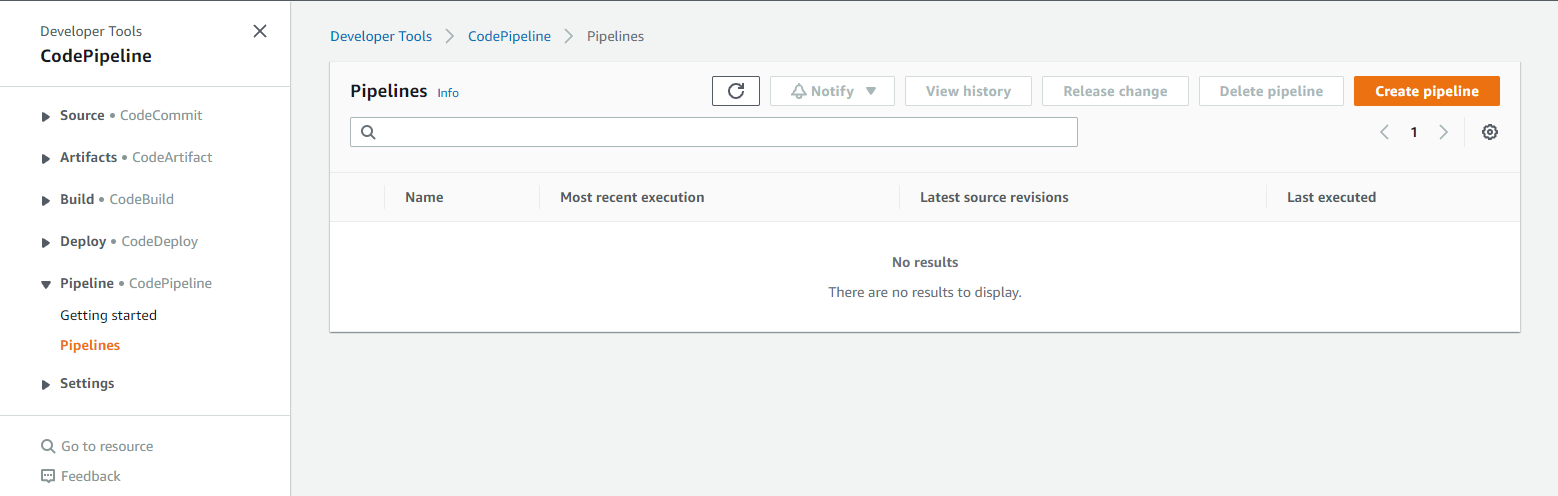
Now it’s time to develop the Lambda function, store the code in AWS CodeCommit, build it, run tests, and deploy it to AWS Lambda. The first thing is to create a pipeline to add the Lambda function.
On the CodePipeline console, click Create Pipeline. A page called “Choose pipeline settings” opens.
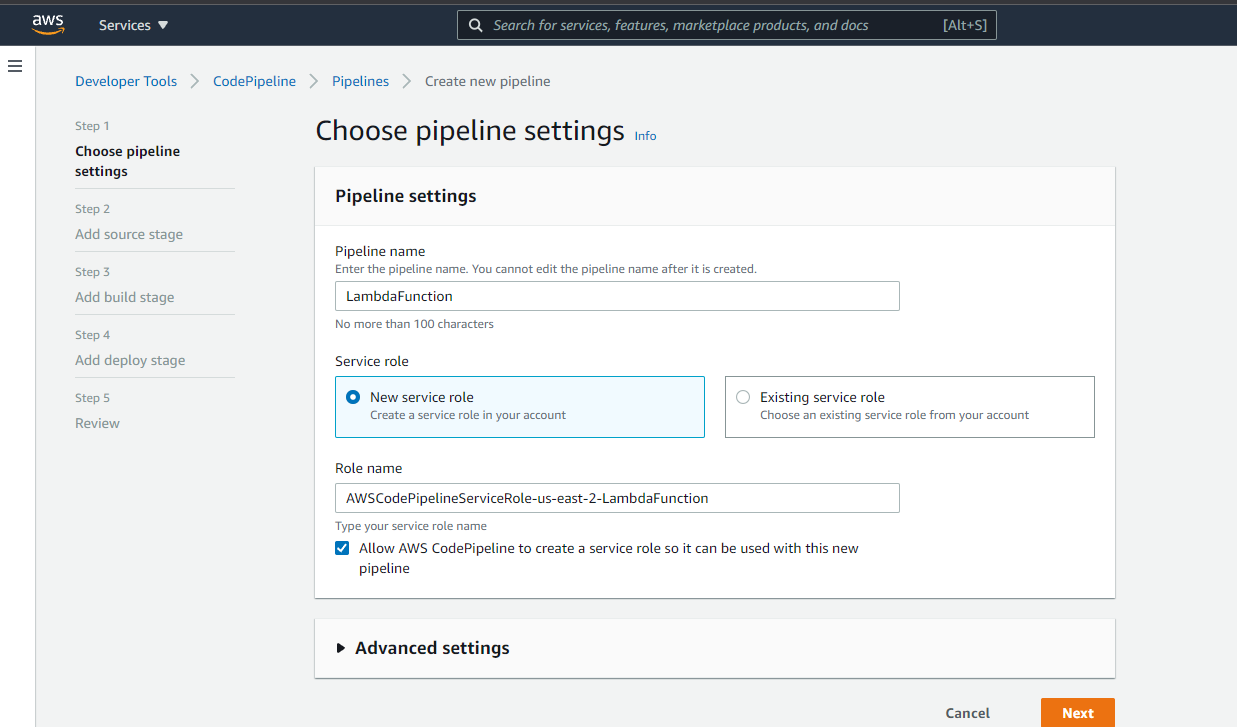
In the Pipeline name field, type “LambdaFunction,” and click Next. This opens the Add source page to choose a source provider.
In the Source provider field, select AWS CodeCommit from the menu.
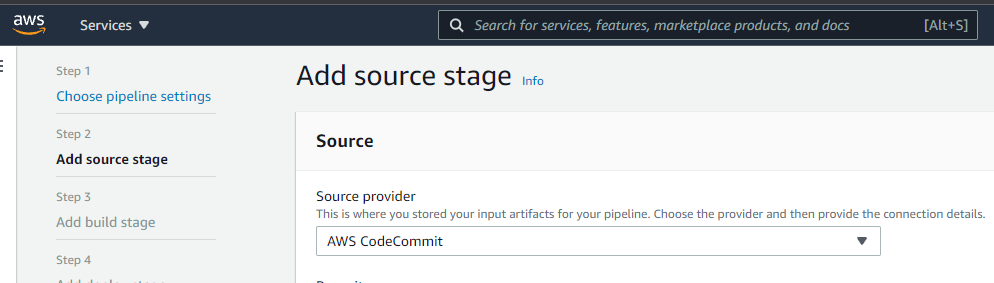
Now, let’s create our lambda function using the AWS CodeCommit console and store the code in AWS CodeCommit.
Open the CodeCommit console. On the top right corner of the page, click Create repository. On the Create repository page, in the Repository name field, type “AWSLambdaFunction.” Enable the Enable Amazon CodeGuru Reviewer for Java and Python option, and click Create. You should see a success message, like so:
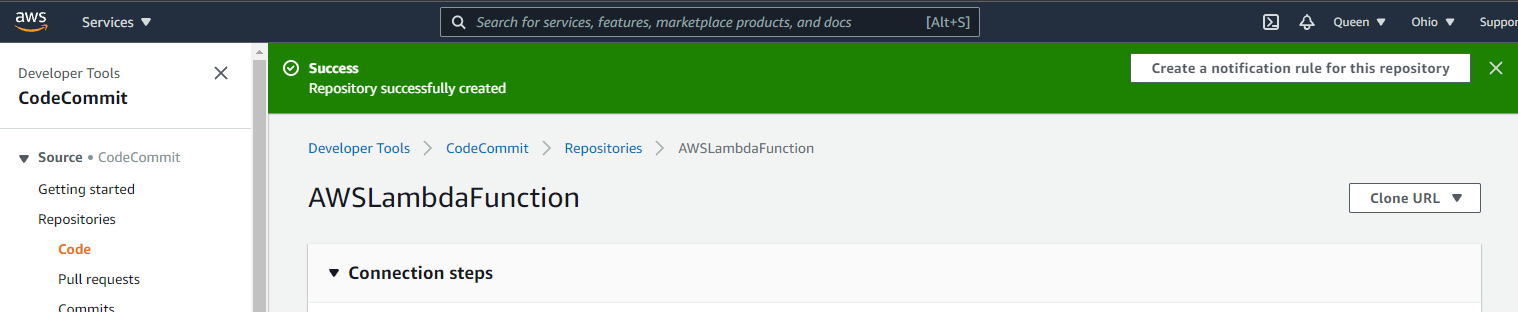
Next, let’s write our Lambda function in our repository. We’ll build a simple Hello.jav function for this purpose.
On the current page, scroll down to the end and click Create File. Copy the code below into the repository and commit the changes to main. Complete the mandatory fields. For the file name, type “Hello.java.” For the author name, type your name. For the email address, type your email address. Type a commit message, and then commit your changes to the repo.
package com.example.lambda.demo;
import com.amazonaws.services.lambda.runtime.Context;
import com.amazonaws.services.lambda.runtime.RequestHandler;
public class Hello implements RequestHandler<Object, String> {
@Override
public String handleRequest(String input, Context context) {
context.getLogger().log("Input: " + input);
String output = "Hello, " + input + "!";
return output;
}
}
Back on the Add Source page, in the Source provider field, select AWS CodeCommit. In the Repository name field, type the name of the repository, “AWSLambdaFunction.” In the Branch name field, type “main” and click Next.
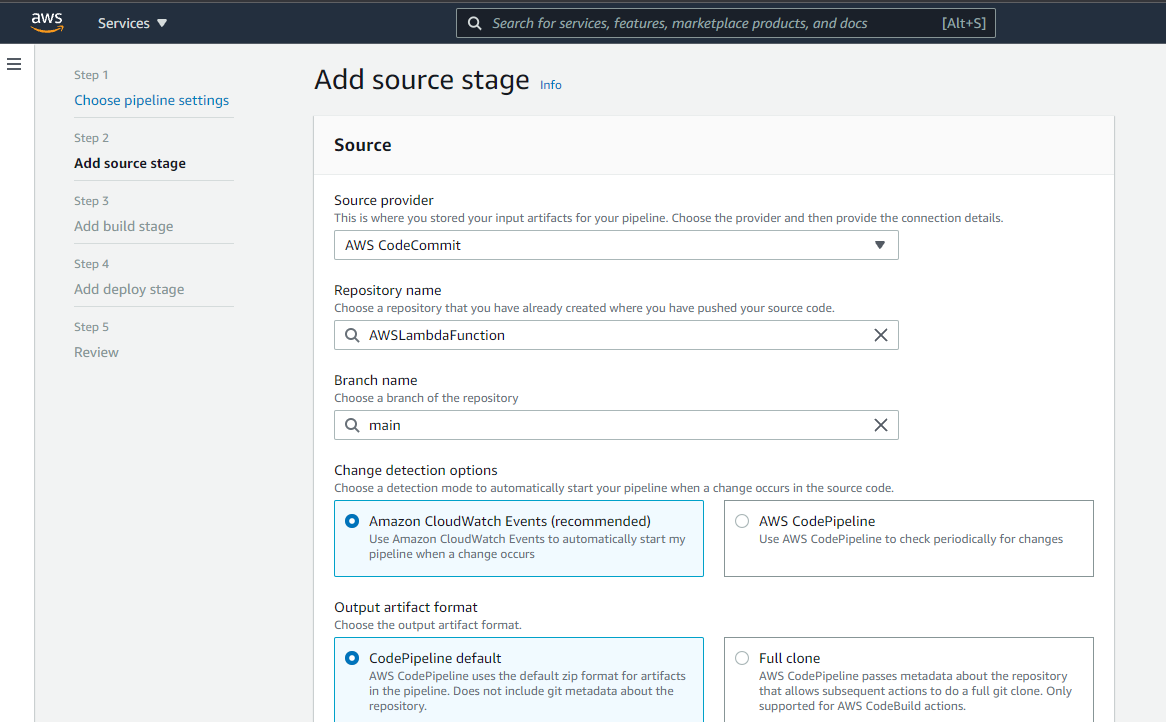
Now, it’s time for us to build our code. The next page is the Add build stage page.
In the Build area, select AWS CodeBuild. In the Project name field, click Create Project and complete the following fields as follows:
- For Project name, type “AWSLambdaFunction.”
- For Environment image, select Managed image.
- For the Operating system, select Windows Server 2019.
- For Image, select aws/codebuild/windows-base:2019-1.0.
- For Service role, select New service role.
- For Buildspec, select Use a buildspec file.
Finally, click Continue to CodePipeline. You should see a success message.
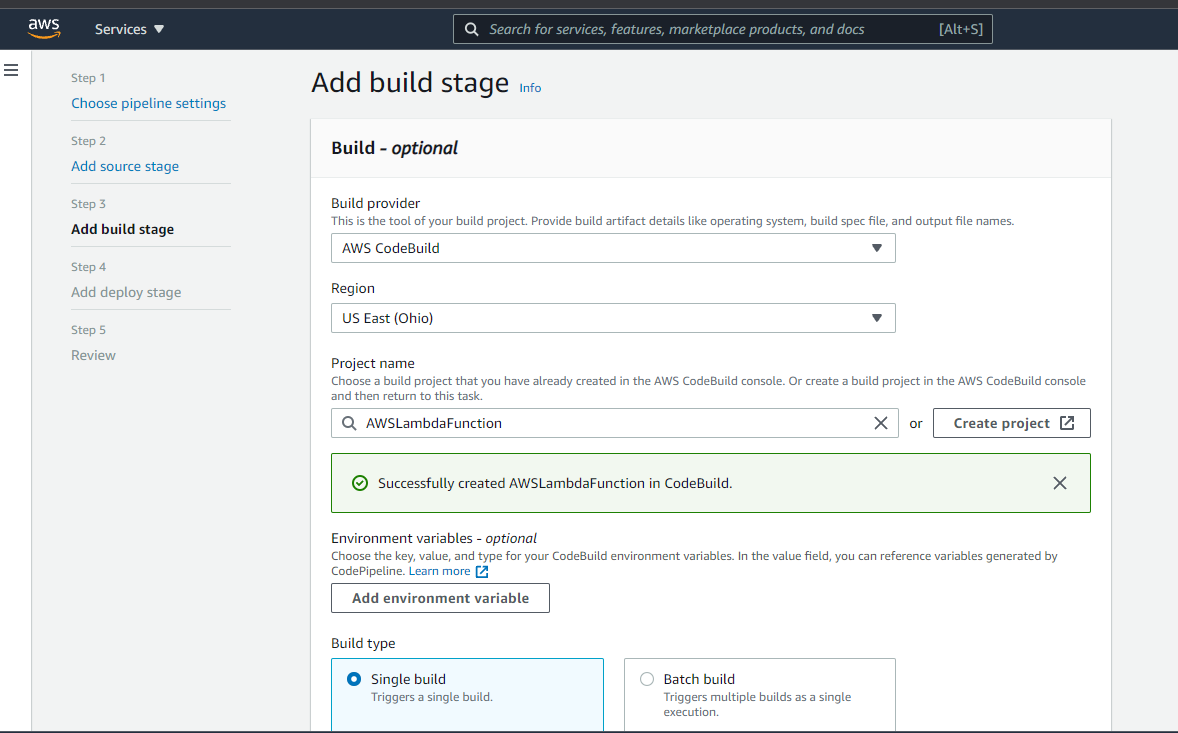
Now it's time for us to deploy our function.
On the Add build stage page, click Next and then deploy. You’ll see a success message.

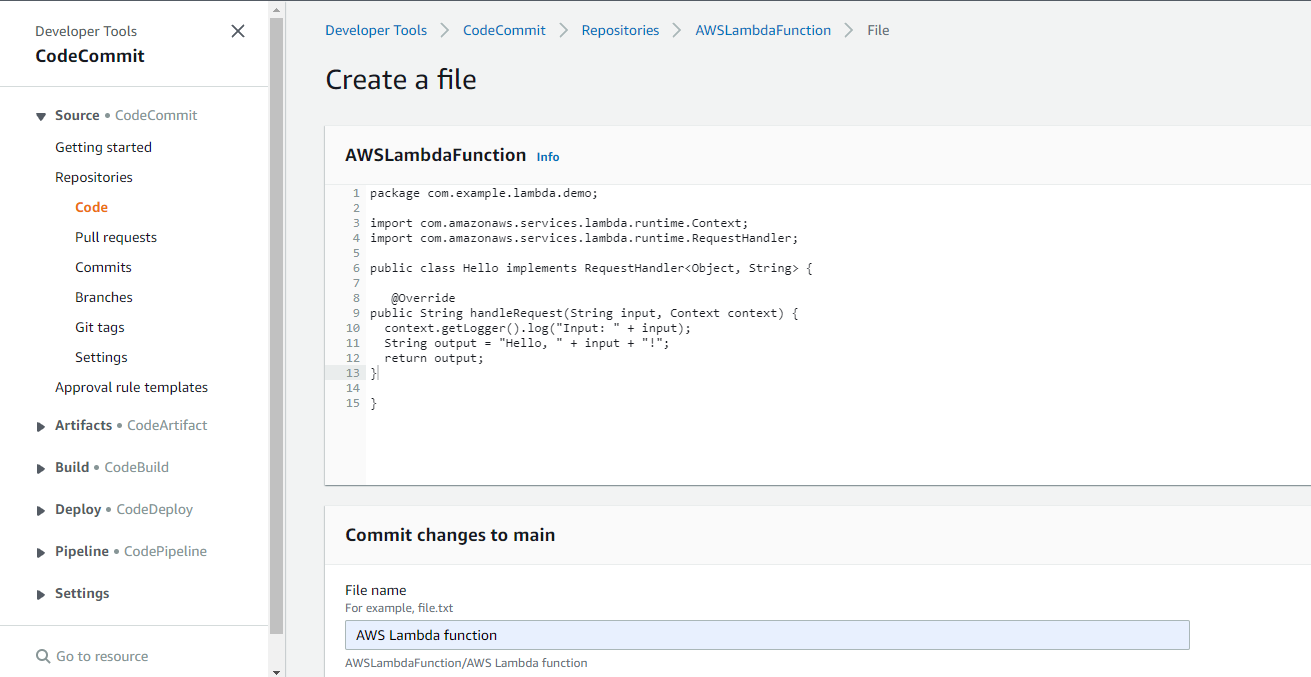
Conclusion
AWS CodePipeline is an automated CI/CD pipeline that takes code from build to test to production automatically. This eases the burden of microservices because there’s no need to deploy each service manually. Once you set up a pipeline, you can commit code and be confident that it will end up in production.
With an automated continuous delivery service, you can deliver innovations to your customers faster and more efficiently.
Author Spotlight:
Ben Hundley
Keep Up To Date With AWS News
Stay up to date with the latest AWS services, latest architecture, cloud-native solutions and more.
Related Blog Posts
Category:
Category:
Category: